nodejs에서 esm과 commonjs 방식의 http server code입니다.
nodejs는 웹서버(http server)가 없습니다.코드로 웹서버의 기능을 구현합니다.
기본적으로 nodejs는 포트번호 3000번을 사용합니다 .
그래서 nodejs패키지인 react나 express만으로 웹사이트를 만든다면
리버스 프록시기능이 있는 Nginx가 좋은 조합이 되리라 생각됩니다.
This is ESM and commonjs style http server code in nodejs.
nodejs does not have a web server (http server). It implements the functions of a web server through code.
By default, nodejs uses port number 3000.
So, if you create a website with only the nodejs package react or express,
I think Nginx with reverse proxy function would be a good combination.
1.ESM,COMMONJS
– nodejs에서는 모듈사용 방식으로 ESM과 COMMONJS라는 방식이 있습니다.
– ESM이 개선된 방식이고 COMMONJS가 이전 방식 입니다.
In nodejs, there are two ways to use modules: ESM and COMMONJS.
ESM is an improved method and COMMONJS is the previous method.
1)ESM
– 파일 확장자가 mjs입니다.
– js파일을 esm방식으로 사용할 경우 package.json파일에서 “Type”:”module”, 을 추가합니다.
– 아래의 코드에서 강조된 녹색부분이 ESM 방식의 코드 입니다.
– 보라색 코드 대신 노란색 코멘트 처리된 코드를 사용해도 됩니다.
-The file extension is mjs.
-When using js files in esm format, add “Type”:”module”, in the package.json file.
-The green part highlighted in the code below is the ESM type code.
-You can use the yellow commented code instead of the purple code.
// server.mjs --> ESM TYPE
import { createServer } from 'node:http';
const server = createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('ESM:Hello World!\n');
});
// starts a simple http server locally on port 3000
/*
server.listen(3000, '127.0.0.1', () => {
console.log('ESM:Listening on 127.0.0.1:3000');
});
*/
const PORT = process.env.PORT || 3000;
server.listen(PORT, () => {
console.log(`ESM:Server is running on port ${PORT}`);
});
-위의 코드를 server.mjs파일이 아닌 server.js파일로 사용하기 원한다면 package.json파일에 ‘ “Type”:”module”, ‘을 다음과 같이 추가합니다.
-package.json파일은 command line에서 npm명령으로 모듈을 설치하면 자동으로 생성됩니다.
-If you want to use the above code in a server.js file rather than a server.mjs file, add ‘ “Type”:”module”, ‘ to the package.json file as follows.
-The package.json file is automatically created when you install the module using the npm command from the command line.
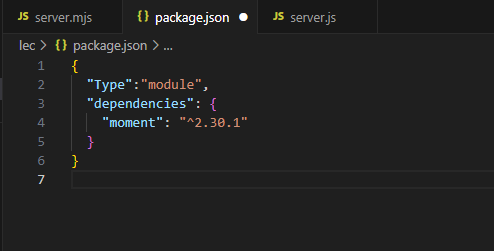
2)COMMONJS
-파일확장자가 js입니다.
-nodejs에서 제공하는 기본적인 모듈 사용방식입니다. 특별한 셋팅이 필요 하지 않습니다.
-아래의 코드에서 강조된 녹색부분이 COMMONJS 방식의 코드 입니다.
-보라색 부분은 COMMONJS를 사용해서 바뀐 부분입니다.
-The file extension is js.
-This is the basic module usage method provided by nodejs. No special settings are required.
The purple part is the part that was changed using COMMONJS.
//server.js --> Commonjs type
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('CommonJS:Hello World!\n');
});
// Start the server
const PORT = process.env.PORT || 3000;
server.listen(PORT, () => {
console.log(`CommonJS:Server is running on port ${PORT}`);
});