-탭 네비게이터를 사용하는 방법에 대한 설명입니다.
Here’s an explanation of how to use the tab navigator.
-탭 네비게이터는 앱에서 사용가능한 메뉴의 한 종류 입니다.
Tab navigator is a type of menu available in apps.
-탭 네비게이터는 앱의 하단에 탭을 할때 화면 전환을 할 수 있도록 도와줍니다.
The tab navigator helps you switch screens when you tap at the bottom of the app.
-탭 네비게이터의 모양은 아래 이미지와 같습니다.
The tab navigator will look like the image below.
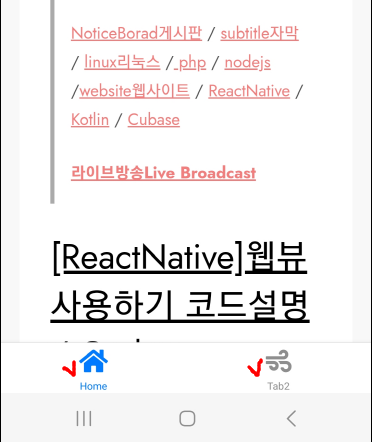
-아래는 앱의 실행 화면입니다.
Below is the app launch screen.
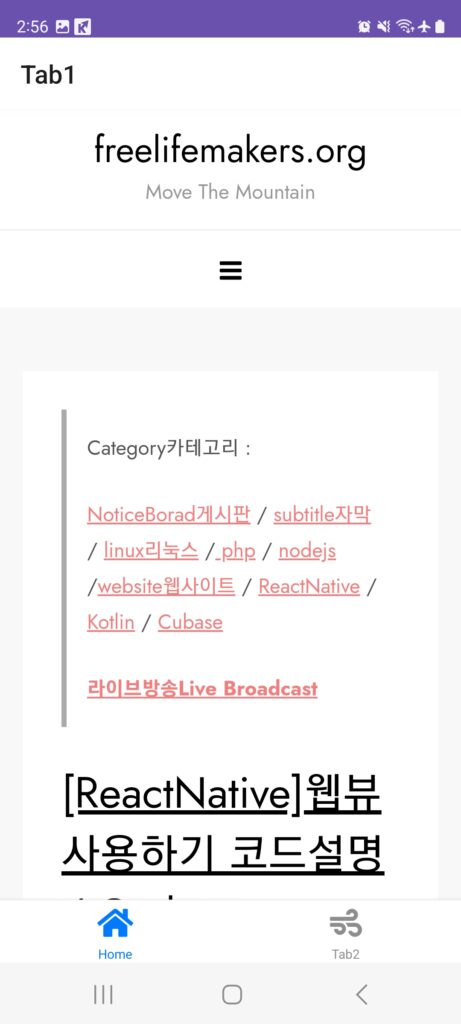
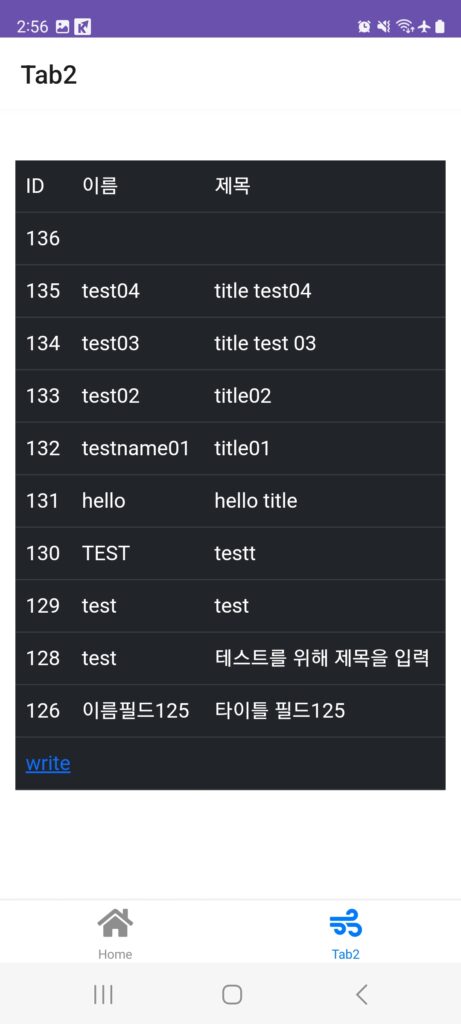
1.모듈설치(install modules)
- 탭 네비게이터를 사용하기 위해서는 모듈을 설치해야 합니다
To use the tab navigator, you need to install the module
- 탭 네비게이터를 위해 설치해야 하는 모듈은 다음과 같습니다.
The modules you need to install for the tab navigator are:
@react-navigation/bottom-tabs
@react-navigation/native
react-native-safe-area-context
react-native-screens
- @react-navigation/bottom-tabs 이 모듈이 탭 네비게이터 모듈입니다.
“@react-navigation/bottom-tabs ” This module is the tab navigator module.
- 나머지 모듈은 탭 네비게이터와 직접 관련이 없지만 설치 하지 않으면 오류가 발생합니다.
The remaining modules are not directly related to the tab navigator, but will cause errors if you don’t install them.
- 그래서 나머지 모듈도 반드시 설치해야 합니다.
So, you must install the remaining modules as well.
- 탭네비게이터에는 아이콘이 사용됩니다. 아이콘을 위한 모듈도 설치 합니다.
Icons are used in the tab navigator. Also install modules for icons.
react-native-vector-icons
- 이 앱을 실행하기 위해서 사용되는 전체 모듈 입니다.
This is the entire module used to run this app.
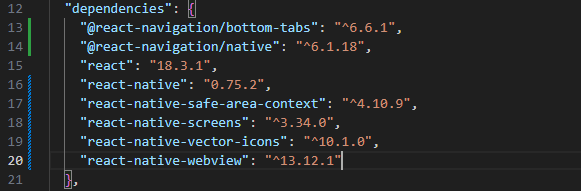
2.코드 작성 / Write code
-이 앱의 디렉토리 구조입니다.
This is the directory structure for this app
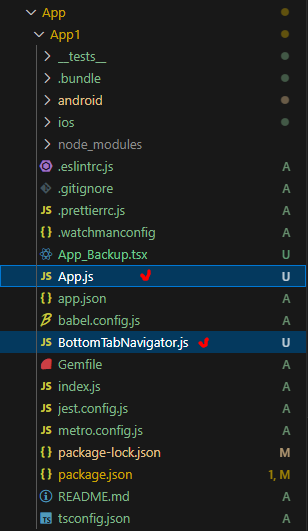
-App.js파일에 다음 코드를 작성 합니다.
Write the following code in the App.js file.
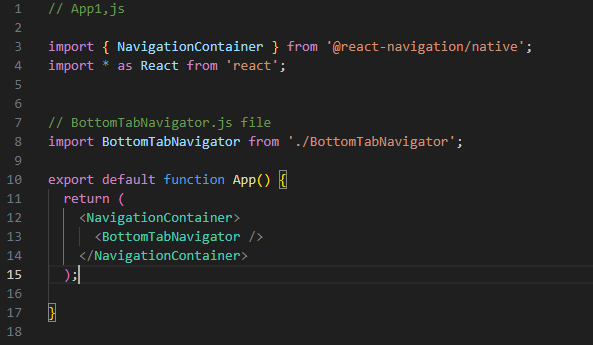
-BottomTabNavigator.js파일을 만들고 다음 코드를 작성합니다.
Create a BottomTabNavigator.js file and write the following code.
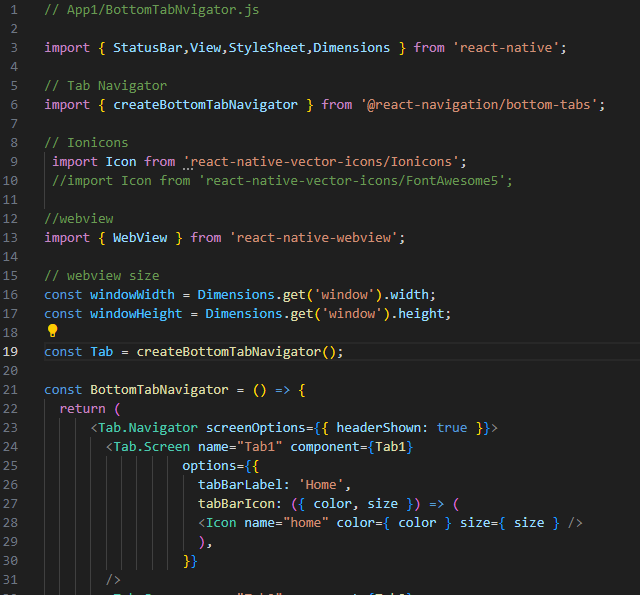
4.npm run android
-npm run android명령으로 디버깅을 실행합니다.
Execute debugging with the “npm run android” command.
5.전체 코드(full code)
-이번 포스트에서 설명하지 못한 부분은 다음 포스트에서 설명하겠습니다.
Anything that was not explained in this post will be explained in the next post.
// App.js
import { NavigationContainer } from '@react-navigation/native';
import * as React from 'react';
// BottomTabNavigator.js file
import BottomTabNavigator from './BottomTabNavigator';
export default function App() {
return (
<NavigationContainer>
<BottomTabNavigator />
</NavigationContainer>
);
}
// BottomTabNvigator.js
import { StatusBar,View,StyleSheet,Dimensions } from 'react-native';
// Tab Navigator
import { createBottomTabNavigator } from '@react-navigation/bottom-tabs';
// Ionicons
import Icon from 'react-native-vector-icons/Ionicons';
//import Icon from 'react-native-vector-icons/FontAwesome5';
//webview
import { WebView } from 'react-native-webview';
// webview size
const windowWidth = Dimensions.get('window').width;
const windowHeight = Dimensions.get('window').height;
const Tab = createBottomTabNavigator();
const BottomTabNavigator = () => {
return (
<Tab.Navigator screenOptions={{ headerShown: true }}>
<Tab.Screen name="Tab1" component={Tab1}
options={{
tabBarLabel: 'Home',
tabBarIcon: ({ color, size }) => (
<Icon name="home" color={ color } size={ size } />
),
}}
/>
<Tab.Screen name="Tab2" component={Tab2}
options={{
tabBarLabel: 'Tab2',
tabBarIcon: ({ color, size }) => (
<Icon name="bag-add" color={ color } size={ size } />
),
}}
/>
</Tab.Navigator>
);
}
const Tab1 = () => {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<WebView style={styles.webview}
source={{ uri: 'https://freelifemakers.org' }}
allowsFullscreenVideo={true}
allowsInlineMediaPlayback={true}
javaScriptEnabled={true}
scalesPageToFit={true}
domStorageEnabled={true}
mediaPlaybackRequiresUserAction={false}
/>
<StatusBar
barStyle="light-content" // Options: 'default', 'light-content', 'dark-content'
backgroundColor="#6a51ae" // For Android
hidden={false} // Show or hide the status bar
translucent={true} // Allow content to render under the status bar
/>
</View>
);
}
const Tab2 = () => {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<WebView style={styles.webview}
source={{ uri: 'https://freelifemakers.org/lec/list01.php' }}
allowsFullscreenVideo={true}
allowsInlineMediaPlayback={true}
javaScriptEnabled={true}
scalesPageToFit={true}
domStorageEnabled={true}
mediaPlaybackRequiresUserAction={false}
/>
<StatusBar
barStyle="light-content" // Options: 'default', 'light-content', 'dark-content'
backgroundColor="#6a51ae" // For Android
hidden={false} // Show or hide the status bar
translucent={true} // Allow content to render under the status bar
/>
</View>
);
}
// webview style
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
//justifyContent: 'space-between',
justifyContent: 'center',
},
// webview size
webview: {
flex: 1,
width: windowWidth,
height: windowHeight,
},
});
export default BottomTabNavigator