회원 가입된 사용자의 비밀번호가 의도치 않게 노출될 수 있습니다
Passwords of registered users may be exposed unintentionally..
그래서 사용자의 개인 정보가 해킹되거나 악용되는 것을 방지하기 위해서 비밀번호를 암호화 시키는게 중요합니다.
Therefore, it is important to encrypt passwords to prevent hacking and misuse of user passwords.
아래는 bcrpt모듈을 사용해서 사용자의 비밀번호를 암호화 하는 방법입니다.
Below is how to encrypt your password.
이전에 다룬 내용은 아래의 이전 포스트를 참조하세요
If you would like to check more details, please refer to the previous post.
previous post이전포스트
간단한 회원가입 페이지 만들기 / Simple Create a simple membership registration page
- bcrypt모듈설치(bcrypt module install)
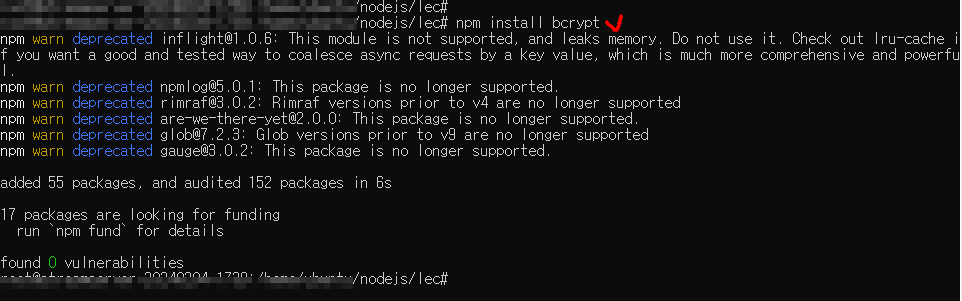
-모듈설치확인(Check module installation)
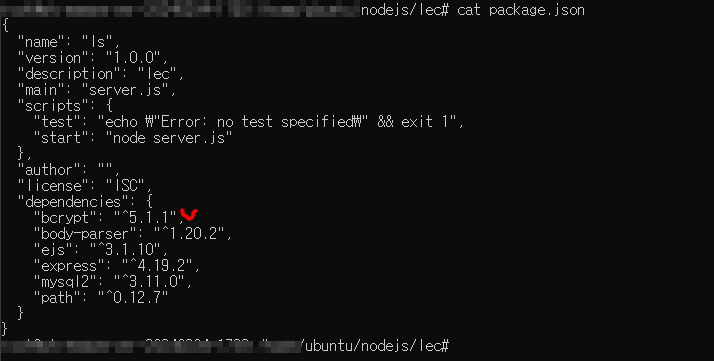
2.모듈 호출하기(Importing module)
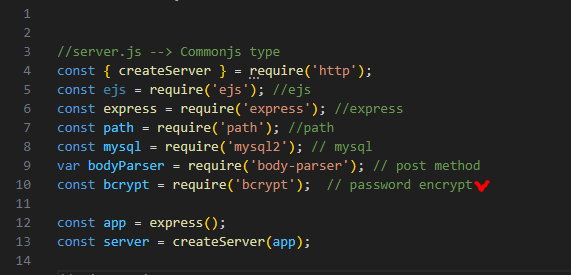
3.패스워드 암호화하기(Encrypt passworrd)
-bcrypt.hashSync(postPassword,10), 이 부분이 사용자 비밀번호(postPassword)를 암호화 시키는 부분입니다.
bcrypt.hashSync(postPassword,10), This is the part that encrypts the usres password.
-10은 암호화 모듈수행 횟수 입니다.
10 is the number of times the encryption module is executed.
-기본적으로 암호해독이 불가능하지만 암호화 모듈 수행 횟수가 너무 적으면 암호화 패턴을 파악하는 사람이 있다고 합니다.
It is said that decryption is basically impossible, but if the encryption module is performed too few times, there are people who can figure out the encryption pattern.
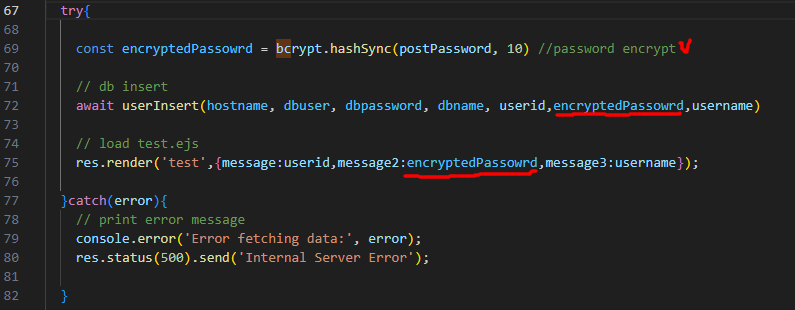
4.브라우저테스트(browser test)

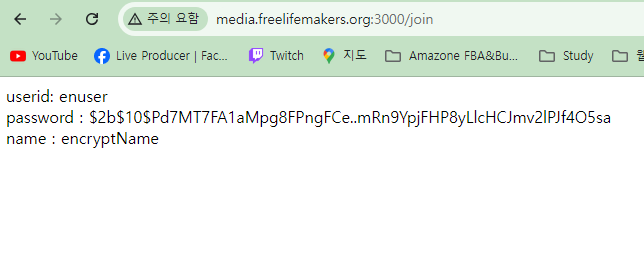
5.Mysql Database
-브라우저와 동일한 패스워드가 저장된 것을 확인 할 수 있습니다.
You can check that the same password as your browser is saved.
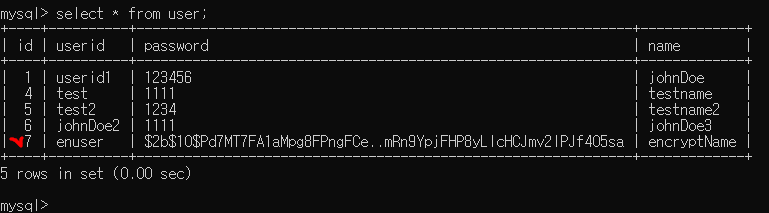
6.server.js전체코드(server.js full code)
//server.js --> Commonjs type
const { createServer } = require('http');
const ejs = require('ejs'); //ejs
const express = require('express'); //express
const path = require('path'); //path
const mysql = require('mysql2'); // mysql
var bodyParser = require('body-parser'); // post method
const bcrypt = require('bcrypt'); // password encrypt
const app = express();
const server = createServer(app);
// ejs settings
//-----------------------------------------------
app.set('view engine','ejs');
app.set('views','./public');
//bodyParser
app.use(bodyParser.urlencoded({ extended: false }));
// Serve static files from the 'public' directory
app.use(express.static(path.join(__dirname, "public")));
//-----------------------------------------------
// mysql db information
const dbname = "your_db_name";
const hostname = "your_host_name";
const dbuser = "your_db_user";
const dbpassword = "your_db_password";
// module import
const {
userInsert,
} = require("./mysqldb");
/*---------------------------------------------------------------------------
member
---------------------------------------------------------------------------*/
// memberInput.ejs 불러오기 / Load login.ejs
app.get("/memberinput", (req, res) => {
res.render('memberInput');
});
// 회원가입 / join the membership
app.post("/join", async(req, res) => {
// post method
var userid = req.body.userid;
var username = req.body.username;
var postPassword = req.body.password;
const connection = mysql.createConnection({
host : hostname,
user : dbuser,
password : dbpassword,
database : dbname
});
try{
const encryptedPassowrd = bcrypt.hashSync(postPassword, 10) //password encrypt
// db insert
await userInsert(hostname, dbuser, dbpassword, dbname, userid,encryptedPassowrd,username)
// load test.ejs
res.render('test',{message:userid,message2:encryptedPassowrd,message3:username});
}catch(error){
// print error message
console.error('Error fetching data:', error);
res.status(500).send('Internal Server Error');
}
});
// Start the server
const PORT = process.env.PORT || 3000;
server.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});